Introduction
Maximal Extractable Value (MEV) is a fascinating concept in the world of decentralized finance (DeFi). It refers to the maximum value that can be extracted from block production in excess of the standard block reward and gas fees by including, excluding, or reordering transactions within a block. This guide will walk you through the steps to create an arbitrage bot on the Ethereum mainnet, enabling you to capitalize on MEV opportunities.
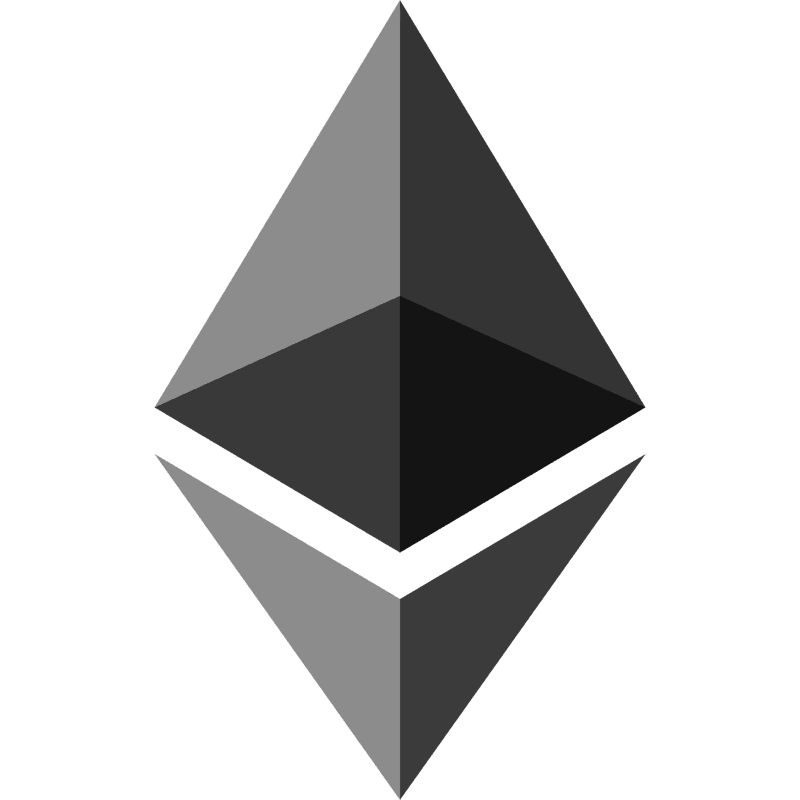
Prerequisites
Before diving into the technical details, make sure you have the following:
- Basic Understanding of Blockchain and Ethereum: Familiarity with how Ethereum works, including smart contracts and transactions.
- Programming Skills: Proficiency in Solidity and Python.
- Development Environment: A setup that includes Node.js, npm, and a Solidity compiler.
- Metamask Wallet: An Ethereum wallet for deploying and interacting with smart contracts.
Step 1: Setting Up Your Development Environment
First, ensure that your development environment is correctly set up. Install Node.js and npm if you haven't already.
sudo apt update
sudo apt install nodejs npm
Next, install Truffle, a development framework for Ethereum.
npm install -g truffle
Step 2: Writing the Arbitrage Smart Contract
Create a new Truffle project and write your smart contract. This contract will handle the logic for finding and executing arbitrage opportunities.
mkdir MEV-Arbitrage-Bot
cd MEV-Arbitrage-Bot
truffle init
In the contracts directory, create a new Solidity file named ArbitrageBot.sol.
pragma solidity ^0.8.0;
contract ArbitrageBot {
address owner;
constructor() {
owner = msg.sender;
}
function executeArbitrage(address tokenA, address tokenB, uint256 amount) public {
require(msg.sender == owner, "Only the owner can execute arbitrage");
// Logic for executing arbitrage goes here
// Example: Swap tokenA for tokenB on one exchange
// and then swap tokenB back to tokenA on another exchange
}
}
Step 3: Deploying the Smart Contract
Compile and deploy your smart contract to the Ethereum mainnet. Make sure to configure your truffle-config.js with the correct network settings.
truffle compile
truffle migrate --network mainnet
Step 4: Creating the Arbitrage Bot
Next, write the Python script that will interact with your smart contract and execute the arbitrage opportunities. Install the necessary libraries.
pip install web3
Create a new Python script named arbitrage_bot.py.
from web3 import Web3
# Connect to Ethereum node
infura_url = "https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID"
web3 = Web3(Web3.HTTPProvider(infura_url))
# Load your smart contract
contract_address = "YOUR_CONTRACT_ADDRESS"
abi = "YOUR_CONTRACT_ABI"
contract = web3.eth.contract(address=contract_address, abi=abi)
# Define the function to find arbitrage opportunities
def find_arbitrage_opportunity():
# Logic to find arbitrage opportunities goes here
pass
# Define the function to execute arbitrage
def execute_arbitrage():
tx = contract.functions.executeArbitrage(tokenA, tokenB, amount).buildTransaction({
'from': web3.eth.defaultAccount,
'gas': 2000000,
'gasPrice': web3.toWei('50', 'gwei')
})
signed_tx = web3.eth.account.signTransaction(tx, private_key='YOUR_PRIVATE_KEY')
tx_hash = web3.eth.sendRawTransaction(signed_tx.rawTransaction)
print(f"Transaction sent: {tx_hash.hex()}")
# Main logic
if __name__ == "__main__":
while True:
opportunity = find_arbitrage_opportunity()
if opportunity:
execute_arbitrage()
Step 5: Running the Bot
Finally, run your Python script to start your MEV arbitrage bot.
python arbitrage_bot.py
Conclusion
Congratulations! You have successfully created and deployed an MEV arbitrage bot on the Ethereum mainnet. This bot continuously scans for arbitrage opportunities and executes profitable trades automatically. Remember, the DeFi space is highly competitive and constantly evolving, so keep learning and improving your strategies to stay ahead.
I hope this guide helps you get started with your MEV arbitrage bot. If you have any questions or need further assistance, feel free to ask!